### necessary ( 필수 ) ###
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
<!-- JQUERY -->
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<!-- JQUERY-UI -->
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.min.js"></script>
<!-- bootstrap.min.css -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css">
<!-- bootstrap-theme.min.css -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap-theme.min.css">
<!-- bootstrap.min.js -->
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/js/bootstrap.min.js"></script>
|
cs |
jquery-ui 는 모달을 드래그 하기 위해 꼭 필요하다.
### Modal CSS ###
1
2
3
|
.modal { top:60px; z-index: unset; position: relative!important; }
.modal-dialog { position: fixed; left: 0; right: 0; top: 100; margin: 70; padding: 10px;}
.modal-backdrop.in{opacity: 0;}
|
cs |
### HTML (EJS) ###
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
|
<button type='button' data-toggle="modal" data-target="#first_modal">첫번쨰 모달 열기</button>
<button type='button' data-toggle="modal" data-target="#second_modal">두번쨰 모달 열기</button>
<button type='button' data-toggle="modal" data-target="#third_modal">세번쨰 모달 열기</button>
<!--first_modal-->
<div class="modal fade bs-example-modal-lg" id="first_modal" tabindex="-1" role="dialog" aria-labelledby="myLargeModalLabel" aria-hidden="true" data-backdrop="static">
<div class="modal-dialog modal-lg" role="document">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span></button>
<ol class="breadcrumb" style='background-color: white;'>
<li><a href="#">모달</a></li>
<li class="active">첫번쨰 모달</li>
</ol>
</div>
</div>
</div>
</div>
<!--second_modal-->
<div class="modal fade bs-example-modal-lg" id="second_modal" tabindex="-1" role="dialog" aria-labelledby="myLargeModalLabel" aria-hidden="true" data-backdrop="static">
<div class="modal-dialog modal-lg" role="document">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span></button>
<ol class="breadcrumb" style='background-color: white;'>
<li><a href="#">모달</a></li>
<li class="active">두번쨰 모달</li>
</ol>
</div>
</div>
</div>
</div>
<!--third_modal-->
<div class="modal fade bs-example-modal-lg" id="third_modal" tabindex="-1" role="dialog" aria-labelledby="myLargeModalLabel" aria-hidden="true" data-backdrop="static">
<div class="modal-dialog modal-lg" role="document">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span></button>
<ol class="breadcrumb" style='background-color: white;'>
<li><a href="#">모달</a></li>
<li class="active">세번쨰 모달</li>
</ol>
</div>
</div>
</div>
</div>
|
cs |
1. 각 모달의 id 값을 설정해준다. 필자는 first_modal, second_modal, third_modal 이렇게 3개의 모달을 준비함.
2. button에 data-toggle="modal" data-target="#first_modal" 구문을 추가한다. data-target="#first_modal"처럼 각 모달의 id 값을 넣어준다.
3. data-backdrop="static" 와 같이 static 값을 넣어주면 모달 영역 밖을 클릭했을때 모달이 닫히지 않는다.
### Modal Draggable & Priority when clicking modal ###
*모달 드래그 방법
*여러개의 모달이 열려있을 경우 사용자가 클릭한 모달이 화면 가장 앞으로 오게 하는 방법.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
|
// 모달 위치 초기화 - 모달 창이 열리기 전에 실행
$(document).on('show.bs.modal', '.modal', function(){
// 화면에 보여지는 모달수 추적
if ( typeof( $('body').data( 'fv_open_modals' ) ) == 'undefined' ) {
$('body').data( 'fv_open_modals', 0 );
}
// 이 모달의 z-index 속성이 정해져 있다면 무시
if ($(this).hasClass('fv-modal-stack')) {
return;
}
$(this).addClass('fv-modal-stack');
$('body').data('fv_open_modals', $('body').data('fv_open_modals' ) + 1 );
$(this).css('z-index', 1040 + (10 * $('body').data('fv_open_modals' )));
$('.modal-backdrop').not('.fv-modal-stack').css('z-index', 1039 + (10 * $('body').data('fv_open_modals')));
$('.modal-backdrop').not('fv-modal-stack').addClass('fv-modal-stack');
// 모달 위치 초기화
$(this).find($('.modal-dialog')).css('top',100);
$(this).find($('.modal-dialog')).css('right',0);
$(this).find($('.modal-dialog')).css('left',0);
// 모달창 드래그 기능
$(this).find($('.modal-dialog')).draggable({ handle: ".modal-header" });
})
// 모달창이 완전히 사라진 후 호출
$(document).on('hidden.bs.modal', '.modal', function(e){
$(this).removeClass( 'fv-modal-stack' );
$('body').data( 'fv_open_modals', $('body').data( 'fv_open_modals' ) - 1 );
});
// 클릭한 모달의 화면 우선순위
$(document).on('click', '.modal', function(){
$(this).css('z-index', 1040 + (10 * $('body').data('fv_open_modals' )));
$('body').data('fv_open_modals', $('body').data('fv_open_modals' ) + 1 );
if ( typeof( $('body').data( 'fv_open_modals' ) ) == 'undefined' ) {
$('body').data( 'fv_open_modals', 0 );
}
});
|
cs |
### RESULT ###
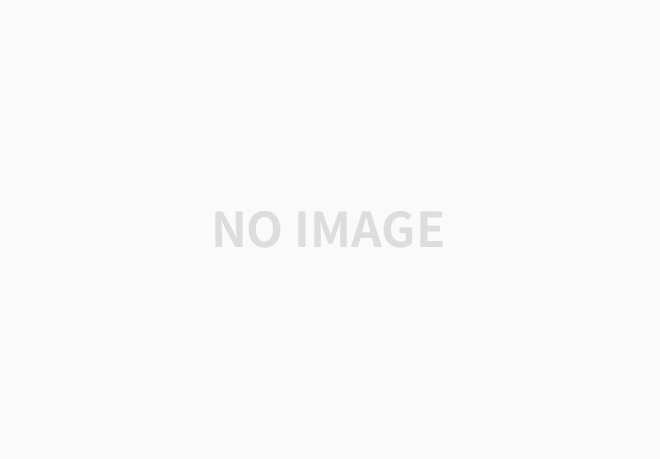
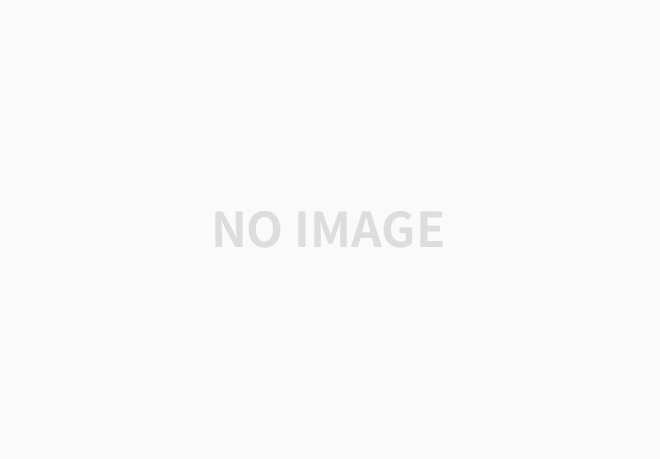
'웹 프로그래밍 > HTML,CSS,JS' 카테고리의 다른 글
javascript 자주쓰는 정규식 표현 모음 (0) | 2020.08.24 |
---|---|
크롬의 alert창 따라하기 (feat. dialog) (0) | 2020.04.02 |
openlayers 5 기본기(지도 생성, 마커/팝업 생성) (1) | 2019.07.23 |
브이월드(opanlayers 3) 지도 + WMS(상권 지도) + 다음 로드뷰 (0) | 2019.07.12 |
동적으로 생성된 파일첨부 데이터 ajax로 보내기 + php (0) | 2019.07.08 |